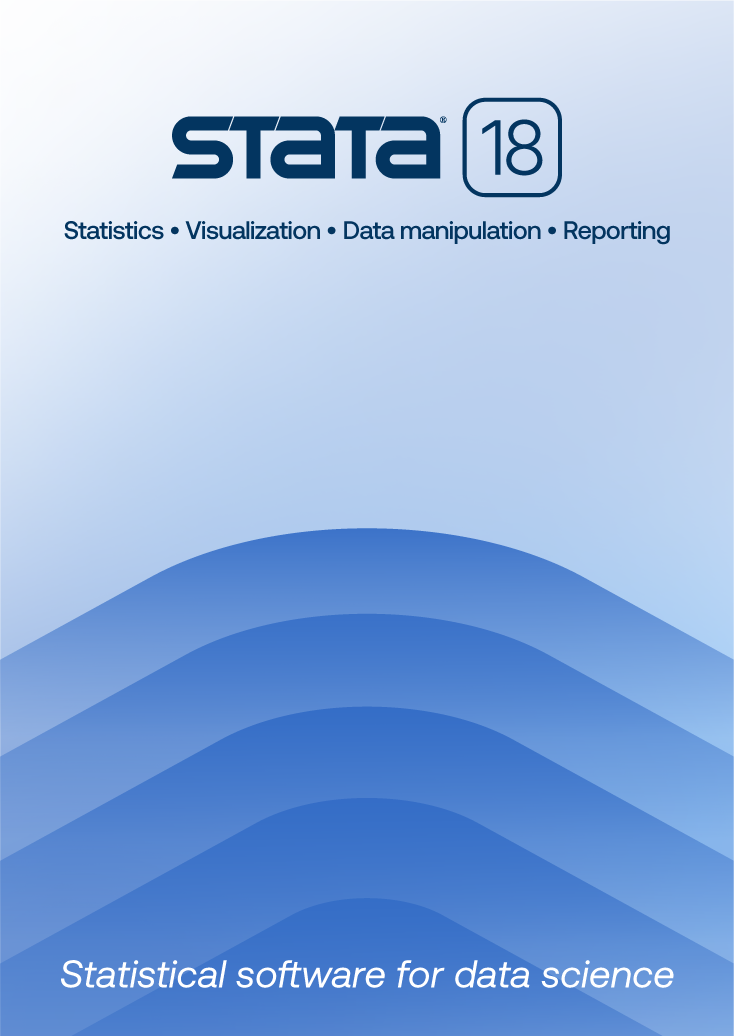
Office Open XML (.docx) files can contain a rich set of formatting properties for paragraphs, text, and tables. This document describes a set of Mata functions to set and modify some formatting properties of .docx files.
The format of Office Open XML file is defined in Standard ECMA-376, which can be found at
http://www.ecma-international.org/publications/standards/Ecma-376.htm
This document references the third edition.
Throughout the document, dh refers to a document ID returned by _docx_new(); tid refers to a table ID returned by _docx_new_table(), _docx_add_matrix(), _docx_add_mata(), or _docx_add_data().
In Office Open XML format, colors are specified in RRGGBB hex string format without a leading # sign, for example, ABF867.
In Office Open XML format, size, length, and width are often measured in twips. A twip is 1/20 of a point, 1/1440 of an inch, or approximately 1/567 of a centimeter.
Any functions that take document ID as an argument may return error code -16514 if the document ID is invalid, or -16513 if the document ID is out of range. Any functions that take table ID as an argument may return error code -16515 if the table ID is out of range, or -16516 if the table ID is invalid. Any functions that take table row number as an argument may return error code -16517 if the row number is out of range. Any functions that take table column number as an argument may return error code -16518 if the column number is out of range. Any functions that reply on the current paragraph may return error code -16519 if there is no current text. Any functions that reply on the current text may return error code -16527 if there is no current text.
Document
The following functions set the properties for a document:
real scalar _docx_set_font(dh, string scalar fontname)
sets the default font for the entire document.
real scalar _docx_set_size(dh, real scalar size)
sets the default text size for the entire document.
real scalar _docx_set_color(dh, string scalar color)
sets the default text color for the entire document. The value of the color is specified in RRGGBB hex format.
real scalar _docx_set_landscape(dh [, real scalar landscape])
sets the document orientation to landscape or portrait. If the optional argument landscape is specified and is 0, the document orientation is set to portrait; otherwise, the document orientation is set to landscape.
real scalar _docx_set_papersize(dh, string scalar paper)
sets the document's paper size. The possible values for paper are letter, legal, A3, A4, and B4JIS.
Paragraph
The following functions set the properties for a paragraph:
real scalar _docx_paragraph_set_font(dh, string scalar fontname)
sets the default font for the text added to the paragraph after the call.
real scalar _docx_paragraph_set_textsize(dh, real scalar size)
sets the default text size for the text added to the paragraph after the call.
real scalar _docx_paragraph_set_color(dh, string scalar color)
sets the default text color for the text added to the paragraph after the call. The value of the color is specified in RRGGBB hex format.
real scalar _docx_paragraph_set_halign(dh, string scalar halignment)
sets the paragraph alignment.
The possible values for halignment are left, right, center, both, and distribute.
Both distribute and both justify text between left and right margins equally, but distributed also changes spacing between words and characters as well.
real scalar _docx_paragraph_set_valign(dh, string scalar valignment)
sets the alignment of characters on each line when the paragraph contains characters of varying size.
The possible values for valignment are auto, baseline, bottom, center, and top.
real scalar _docx_paragraph_set_shading(dh, string scalar fillcolor, color, value)
sets background color, foreground color and fill pattern for the current paragraph.
fillcolor specifies the background color. color specifies the foreground color. val specifies the fill pattern. The possible values are clear (no pattern), pct10, pct12, pct15 . . ., diagCross, diagStripe, horzCross, horzStripe, nil, thinDiagCross, solid, etc.
See ECMA-376, 3rd, § 17.18.78 for a complete listing.
real scalar _docx_paragraph_set_ind(dh, string scalar type, real scalar size)
sets the indentation of the current paragraph.
The possible values for type are left, right, hanging, and firstLine.
Specifying hanging omits the indentation from the first line. Specifying firstLine applies indentation to the first line. If both hanging and firstLine are specified, hanging is used.
size is specified in twips.
real scalar _docx_paragraph_set_spacing(dh, string scalar type, real scalar size)
sets the spacing between lines of text.
The possible values for type are before, after, and line. before specifies the space before the first line of the current paragraph line. after specifies the space after the last line of the current paragraph. line specifies the space between lines within the current paragraph.
size is specified in twips.
real scalar _docx_add_pagebreak(dh)
adds a page break to the document. It ends the current paragraph and the current text. The current paragraph and current text become undefined.
real scalar _docx_paragraph_add_linebreak(dh)
adds a typical line break. New text restarts on the next line.
Text
The following functions change the text properties and applies them to the current text. The current text is set by calling _docx_paragraph_add_text().
real scalar _docx_text_set_font(dh, string scalar fontname)
sets the font for the current text.
real scalar _docx_text_set_color(dh, string scalar color)
sets the color for the current text.
real scalar _docx_text_set_size(dh, real scalar size)
sets the font size for the current text.
real scalar _docx_text_set_bold(dh, real scalar bold)
sets the current text to bold if bold is not 0.
real scalar _docx_text_set_italic(dh, real scalar italic)
sets the current text to italic if italic is not 0.
real scalar _docx_text_set_underline(dh, string scalar pattern)
sets the current text to be underlined. pattern specifies the format of the line. The possible values for pattern are double, single, none, dotted, dottedHeavy, dash, dashedHeavy, dashLong, dashLongHeavy, dotDash, dashDotHeavy, dotDotDash, dashDotDotHeavy, thick, wave, wavyHeavy, wavyDouble, words.
real scalar _docx_text_set_strikeout(dh, real scalar strikeout)
sets the current text to have a strikeout mark if strikeout is not 0.
real scalar _docx_text_set_valign(dh, string scalar valign)
sets the vertical alignment of the current text. The possible values for valign are baseline, subscript, and superscript.
real scalar _docx_text_set_shading(dh, string scalar fill, color, value)
sets the background color, foreground color, and the fill pattern for the text.
fill specifies the background color.
color specifies the foreground color.
val specifies the fill pattern. The possible values are clear (no pattern), pct10, pct12, pct15 . . ., diagCross, diagStripe, horzCross, horzStripe, nil, thinDiagCross, solid, etc. See ECMA-376, 3rd, § 17.18.78 for a complete listing.
real scalar _docx_text_add_cr(dh)
adds a carriage return to the current text.
real scalar _docx_text_add_tab(dh)
adds a tab to the current text. Note that a tab in Word advances to the nearest multiple of the default tab stop.
real scalar _docx_text_add_unicodechar(dh, string scalar value, real scalar ishex)
adds a Unicode character to the current text.
The Unicode character is specified in value as either hex or decimal string. If value is the decimal string representation of the Unicode character, ishex must be set to 0.
It is the programmer's responsibility to set the proper font for the Unicode character to be displayed correctly.
Table
The following functions change the properties of a table:
real scalar _docx_table_set_width(dh, tid, string scalar type, real scalar w)
sets the width of a table.
The possible values for type are auto, dxa, nil, and pct. When type is nil, the table has width 0 and w is ignored. When type is "dxa", the table has width w in twips. When type is pct, the table has the width as a percent of the default table width. The percent is specified in w as fifths of a percent, so 5000 is 100%. The % symbol must not be included.
For example:
_docx_table_set_width(dh, tid, "dxa", 2880) sets the table width to two inches.
_docx_table_set_width(dh, tid1, "pct", 2500) sets the table width to 50% of the default table width.
real scalar _docx_table_set_alignment(dh, tid, string scalar halign)
sets the table alignment. The possible values for halign are left, right, and center.
Note that setting the table alignment has no visible effect if the table has the default width.
real scalar _docx_table_set_ind(dh, tid, string scalar type, real scalar ind)
sets the table indentation. The possible values for type are dxa and nil. If the type is dxa, the table has indentation w in twips. If the type is nil, the table has 0 indentation and w is ignored.
real scalar _docx_table_set_border(dh, tid, string scalar name, val, color)
sets the borders of a table.
The possible values for name are top, bottom, start, end, indiseH, and insideV.
The possible values for val are: single, dashDotStroked, dashed, dashSmallGap, dotDash, dotDotDash, dotted, double, doubleWave, inset, nil, none, outset, thick, thickThinLargeGap, thickThinMediumGap, thickThinSmallGap, thinThickLargeGap, thinThickMediumGap, thinThickSmallGap, thinThickThinLargeGap, thinThickThinMediumGap, thinThickThinSmallGap, threeDEmboss, threeDEngrave, triple, and wave.
For a detailed description of each value, see ECMA-376, 3rd Edition, §17.18.2.
real scalar _docx_table_set_cellmargin(dh, tid, string scalar name, type, real scalar val)
sets the cell margin of a table.
The possible values for name are top, bottom, left, and right.
The possible values for type are dxa and nil.
val is measured in twips.
real scalar _docx_table_set_cellspacing(dh, tid, string scalar type, real scalar val)
sets the spacing between table cells.
The possible values for type are dxa and nil.
val is measured in twips.
real scalar _docx_table_set_layout(dh, tid, string scalar type)
sets the layout of the table.
The possible values for type are fixed and autofit.
Table Row
The following functions change the properties of a row in a table:
real scalar _docx_row_set_cantsplit(dh, tid, real scalar i)
Call this function so row i cannot be split across multiple pages.
real scalar _docx_row_set_header(dh, tid, real scalar i)
Call this function so row i is repeated at the top of each new page on which the table is displayed. The property can be specified for multiple rows to generate a multirow header. The property will be ignored if the row is not the first row.
real scalar _docx_row_set_halign(dh, tid, real scalar rowno, string scalar type)
sets the alignment of the row specified in rowno. The possible values for type are left, right, and center.
Table cell
The following functions change the properties of a cell in a table:
real scalar _docx_cell_set_textfont(dh, tid, real scalar i, j, string scalar fontname)
sets the font of the text in the cell on the ith row and jth column of the table.
real scalar _docx_cell_set_textcolor(dh, tid, real scalar i, j, string scalar color)
sets the color of the text in the cell on the ith row and jth column of the table.
real scalar _docx_cell_set_textsize(dh, tid, real scalar i, j, size)
sets the size of the text in the cell on the ith row and jth column of the table.
real scalar _docx_cell_set_texthalign(dh, tid, real scalar i, j, string scalar halign)
sets the alignment of the text in the cell on the ith row and jth column of the table.
The possible values for halign are left, right, and center.
real scalar _docx_cell_set_textvalign(dh, tid, real scalar i, j, string scalar valign)
sets the vertical alignment of the text in the cell on the ith row and jth column of the table.
The possible values for valign are top, bottom, and center.
real scalar _docx_cell_set_textbold(dh, tid, real scalar i, j, bold)
sets the text in the cell on the ith row and jth column of the table to be bold if bold is not 0.
real scalar _docx_cell_set_textitalic(dh, tid, real scalar i, j, italic)
sets the text in the cell on the ith row and jth column of the table to be italic if italic is not 0.
real scalar _docx_cell_set_textunderline(dh, tid, real scalar i, j, string scalar pattern)
sets the text in the cell on the ith row and jth column of the table to be underlined. pattern specifies the format of the line. The possible values for pattern are double, single, none, dotted, dottedHeavy, dash, dashedHeavy, dashLong, dashLongHeavy, dotDash, dashDotHeavy, dotDotDash, dashDotDotHeavy, thick, wave, wavyHeavy, wavyDouble, words.
real scalar _docx_cell_set_textstrikeout(dh, tid, real scalar i, j, strikeout)
sets the text in the cell on the ith row and jth column of the table to have a strikeout mark if strikeout is not 0.
real scalar _docx_cell_set_border(dh, tid, real scalar i, j, string scalar name, val, color)
sets the border style and color for the cell on the ith row and jth column of the table.
The possible values for name are top, bottom, start, and end.
The possible values for val are: single, dashDotStroked, dashed, dashSmallGap, dotDash, dotDotDash, dotted, double, doubleWave, inset, nil, none, outset, thick, thickThinLargeGap, thickThinMediumGap, thickThinSmallGap, thinThickLargeGap, thinThickMediumGap, thinThickSmallGap, thinThickThinLargeGap, thinThickThinMediumGap, thinThickThinSmallGap, threeDEmboss, threeDEngrave, triple, and wave.
For a detailed description of each value, see ECMA-376, 3rd Edition, §17.18.2.
real scalar _docx_cell_set_shading(dh, tid, real scalar i, j, string scalar fillcolor, color, value)
sets the background color, foreground color, and fill pattern for the cell on the ith row and jth column of the table
fillcolor specifies the background color.
color specifies the foreground color.
val specifies the fill pattern. The possible values are clear (no pattern), pct10, pct12, pct15. . . ., diagCross, diagStripe, horzCross, horzStripe, nil, thinDiagCross, solid, etc.
See ECMA-376, 3rd, § 17.18.78 for a complete listing.
Example 1: Font, size, and color properties
This example shows how to change text font, size, and color properties using different functions.
mata: dh = _docx_new() // set default document font, size, color, and orientation _docx_set_font(dh, "Castellar") _docx_set_size(dh, 144) _docx_set_color(dh, "691F01") _docx_set_landscape(dh) // add a new paragraph with center alignment and document // default properties _docx_paragraph_new(dh, "Stata/MP") _docx_paragraph_set_halign(dh, "center") // overwrite document font, size, and color with paragraph properties _docx_paragraph_new(dh, "") _docx_paragraph_set_textsize(dh, 64) _docx_paragraph_set_font(dh, "Arial") _docx_paragraph_set_color(dh, "0000FF") _docx_paragraph_add_text(dh, "From dual-core laptops to the big iron") _docx_paragraph_add_text(dh, " of multiprocessor servers, Stata") _docx_paragraph_add_text(dh, " gets the most out of multicore systems.") // overwrite paragraph font, size, and color with text properties _docx_paragraph_add_text(dh, " No other statistical software comes close.") _docx_text_set_size(dh, 96) _docx_paragraph_set_font(dh, "Courier New") _docx_text_set_color(dh, "BC05DF") _docx_save(dh, "example1.docx", 1) _docx_close(dh) end
Example 2: Paragraph shading
This example shows how to change paragraph background color, foreground color, and fill pattern properties.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh, "Set paragraph shading", "Title") _docx_paragraph_new(dh,"") _docx_paragraph_set_shading(dh, "FFFF00", "FF0000", "pct25") _docx_paragraph_add_text(dh," Shading pct25, fill FFFF00, and color FF0000.") _docx_paragraph_new(dh,"") _docx_paragraph_set_shading(dh, "FF0000", "0000FF", "pct50") _docx_paragraph_add_text(dh," Shading pct50, fill FF0000, and color 0000FF.") _docx_paragraph_new(dh,"") _docx_paragraph_set_shading(dh, "FF8800", "8888FF", "solid") _docx_paragraph_add_text(dh," Shading solid, fill FF8800, and color 8888FF.") _docx_save(dh, "example2.docx", 1) _docx_close(dh) end
Example 3: Paragraph indentation
This example shows how to change paragraph indentation properties.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh, "Set paragraph indentation", "Title") _docx_paragraph_new(dh,"") _docx_paragraph_set_ind(dh, "left", 1440) _docx_paragraph_set_ind(dh, "right", 2880) _docx_paragraph_add_text(dh,"Set paragraph indentation to 1440 twips") _docx_paragraph_add_text(dh," from left and 2880 twips from right,") _docx_paragraph_add_text(dh," i.e., 1 inch form left and 2 inches from right.") _docx_paragraph_new(dh,"") _docx_paragraph_set_ind(dh, "left", 2880) _docx_paragraph_set_ind(dh, "right", 1440) _docx_paragraph_add_text(dh, "Set indentation to 2880 twips") _docx_paragraph_add_text(dh, " from left and 1440 twips from right.") _docx_save(dh, "example3.docx", 1) _docx_close(dh) end
Example 4: Paragraph alignment
This example shows how to change paragraph alignment properties.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh, "Set paragraph alignment", "Title") _docx_paragraph_new(dh,"") _docx_paragraph_set_halign(dh, "left") _docx_paragraph_add_text(dh,"Set paragraph to be left adjusted.") _docx_paragraph_new(dh,"") _docx_paragraph_set_halign(dh, "right") _docx_paragraph_add_text(dh,"Set paragraph to be right adjusted.") _docx_paragraph_new(dh,"") _docx_paragraph_set_halign(dh, "distribute") _docx_paragraph_add_text(dh, "Set paragraph alignment to be distribute,") _docx_paragraph_add_text(dh, " which justifies text between both margins") _docx_paragraph_add_text(dh, " equally, and justifies space between") _docx_paragraph_add_text(dh, " words and characters.") _docx_paragraph_new(dh,"") _docx_paragraph_set_halign(dh, "both") _docx_paragraph_add_text(dh, "Set paragraph alignment to be both, which") _docx_paragraph_add_text(dh, " justifies text between both margins") _docx_paragraph_add_text(dh, " equally, but not the space between characters.") _docx_save(dh, "example4.docx", 1) _docx_close(dh) end
Example 5: Paragraph vertical alignment
This example shows how to change paragraph vertical alignment properties.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh, "Set paragraph vertical alignment", "Title") _docx_paragraph_new(dh,"") _docx_paragraph_set_valign(dh, "baseline") _docx_paragraph_set_textsize(dh, 36) _docx_paragraph_add_text(dh,"Text is aligned to the baseline.") _docx_paragraph_set_textsize(dh, 12) _docx_paragraph_add_text(dh, " The quick brown fox jumped over the lazy dog.") _docx_paragraph_new(dh,"") _docx_paragraph_set_valign(dh, "bottom") _docx_paragraph_set_textsize(dh, 36) _docx_paragraph_add_text(dh, "Text is aligned to the bottom.") _docx_paragraph_set_textsize(dh, 12) _docx_paragraph_add_text(dh, " The quick brown fox jumped over the lazy dog.") _docx_paragraph_new(dh,"") _docx_paragraph_set_valign(dh, "center") _docx_paragraph_set_textsize(dh, 36) _docx_paragraph_add_text(dh," Text is aligned to the center.") _docx_paragraph_set_textsize(dh, 12) _docx_paragraph_add_text(dh, " The quick brown fox jumped over the lazy dog.") _docx_paragraph_new(dh,"") _docx_paragraph_set_valign(dh, "top") _docx_paragraph_set_textsize(dh, 36) _docx_paragraph_add_text(dh," Text is aligned to the top.") _docx_paragraph_set_textsize(dh, 12) _docx_paragraph_add_text(dh, " The quick brown fox jumped over the lazy dog.") _docx_save(dh, "example5.docx", 1) _docx_close(dh) end
Example 6: Bold and italic text
This example shows how to set text to bold and italic.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Set text to bold and/or italic", "Title") _docx_paragraph_new(dh,"Text is bold.") // _docx_text_set_bold() sets the current text to bold, which is // "Text is bold" _docx_text_set_bold(dh, 1) _docx_paragraph_add_text(dh," Text is not bold.") // notice _docx_paragraph_add_text() starts a new current text; hence, it does // not inherits the previous text's bold property. _docx_paragraph_add_text(dh," Text is bold again.") _docx_text_set_bold(dh, 1) // set the current text to bold _docx_text_add_text(dh," Text keeps being bold.") // notice _docx_text_add_text() adds text to the current text; hence, // the newly added text inherits the bold property from the current text. _docx_paragraph_new(dh,"Text is italic.") _docx_text_set_italic(dh, 1) _docx_paragraph_add_text(dh," Text is not italic.") _docx_paragraph_add_text(dh," Text is italic again.") _docx_text_set_italic(dh, 1) _docx_paragraph_new(dh,"Text is bold and italic.") _docx_text_set_italic(dh, 1) _docx_text_set_bold(dh, 1) _docx_paragraph_add_text(dh," Text is bold.") _docx_text_set_bold(dh, 1) _docx_paragraph_add_text(dh," Text is italic.") _docx_text_set_italic(dh, 1) _docx_paragraph_add_text(dh," Text is neither bold nor italic.") _docx_save(dh, "example6.docx", 1) _docx_close(dh) end
Example 7: Unicode characters
This example adds Chinese Unicode characters to the document.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Unicode characters (Chinese)", "Title") _docx_paragraph_new(dh, "") _docx_text_set_font(dh, "SimSun") _docx_text_set_size(dh, 72) _docx_text_add_unicodechar(dh, "23398", 0) _docx_text_add_unicodechar(dh, "32780", 0) _docx_text_add_unicodechar(dh, "19981", 0) _docx_text_add_unicodechar(dh, "24605", 0) _docx_text_add_unicodechar(dh, "21017", 0) _docx_text_add_unicodechar(dh, "32596", 0) _docx_text_add_unicodechar(dh, "65292", 0) _docx_text_add_unicodechar(dh, "24605", 0) _docx_text_add_unicodechar(dh, "32780", 0) _docx_text_add_unicodechar(dh, "19981", 0) _docx_text_add_unicodechar(dh, "23398", 0) _docx_text_add_unicodechar(dh, "21017", 0) _docx_text_add_unicodechar(dh, "27526", 0) _docx_paragraph_new(dh, "") _docx_paragraph_set_textsize(dh, 24) _docx_paragraph_add_text(dh, "Learning without thought") _docx_paragraph_add_text(dh, " is labor lost; thought without") _docx_paragraph_add_text(dh, " learning is perilous --") _docx_paragraph_add_text(dh, " Confucian Analects,") _docx_paragraph_add_text(dh, " translated by James Legge.") _docx_save(dh, "example7.docx", 1) _docx_close(dh) end
Example 8: Text vertical alignment (subscript and superscript)
This example shows text vertical alignment, specifically subscript and superscript.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Text vertical alignment", "Title") _docx_paragraph_new(dh, "Vertical") _docx_paragraph_add_text(dh, "subscript") _docx_text_set_valign(dh, "subscript") _docx_paragraph_add_text(dh, " Vertical") _docx_paragraph_add_text(dh, "superscript") _docx_text_set_valign(dh, "superscript") _docx_paragraph_add_text(dh, " Vertical") _docx_paragraph_add_text(dh, "baseline") _docx_text_set_valign(dh, "normal") _docx_save(dh, "example8.docx", 1) _docx_close(dh) end
Example 9: Text shading
This example shows text shading.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Text shading", "Title") _docx_paragraph_new(dh, "") _docx_paragraph_add_text(dh, "The text has fill pattern pct25") _docx_text_add_text(dh, ", fill color FFFF00,") _docx_text_set_shading(dh, "FFFF00", "FF0000", "pct25") _docx_text_add_text(dh, " and background FF0000.") _docx_save(dh, "example9.docx", 1) _docx_close(dh) end
Example 10: Table width
This example shows how to set table width and how to include double quotes in the output text.
local dq = `"""' mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Table width", "Title") temp = J(1, 5, 1) stemp = " "+`"`dq'"' + "dxa" + `"`dq'"' _docx_paragraph_new(dh, "Set table width property to ") _docx_paragraph_add_text(dh, stemp) _docx_paragraph_add_text(dh, " which is measured in twentieths of") _docx_paragraph_add_text(dh, " a point (1/1440 of an inch)") tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_width(dh, tid1, "dxa", 2880) tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_width(dh, tid1, "dxa", 1440) stemp = " "+`"`dq'"' + "pct" + `"`dq'"' _docx_paragraph_new(dh,"Set table width property to") _docx_paragraph_add_text(dh, stemp) _docx_paragraph_add_text(dh, " which is measured as a percent") _docx_paragraph_add_text(dh, " of the default table width.") _docx_paragraph_add_text(dh, " The value w is interpreted as") _docx_paragraph_add_text(dh, " fifths of a percent, so 5000 is 100%.") tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_width(dh, tid1, "pct", 5000) tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_width(dh, tid1, "pct", 2500) stemp = " "+`"`dq'"' + "nil" + `"`dq'"' _docx_paragraph_new(dh,"Set table width property to") _docx_paragraph_add_text(dh, stemp) _docx_paragraph_add_text(dh, " which means a width of zero.") _docx_paragraph_add_text(dh," The value w is ignored.") tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_width(dh, tid1, "nil", 0) tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_width(dh, tid1, "nil", 10000) stemp = " "+`"`dq'"' + "auto" + `"`dq'"' _docx_paragraph_new(dh,"Set table width property to") _docx_paragraph_add_text(dh, stemp) _docx_paragraph_add_text(dh, " which implies that the columns") _docx_paragraph_add_text(dh, " in a table automatically") _docx_paragraph_add_text(dh, " fit the contents.") _docx_paragraph_add_text(dh," The value w is ignored.") tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_width(dh, tid1, "auto", -1) tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_width(dh, tid1, "auto", 0) _docx_save(dh, "example10.docx", 1) _docx_close(dh) end
Example 11: Table alignment
This example shows how to set the table alignment.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Table alignment", "Title") temp = J(1, 5, 1) tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_width(dh, tid1, "dxa", 2880) _docx_table_set_alignment(dh, tid1, "right") tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_width(dh, tid1, "dxa", 2880) _docx_table_set_alignment(dh, tid1, "center") tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_width(dh, tid1, "dxa", 2880) _docx_table_set_alignment(dh, tid1, "left") _docx_save(dh, "example11.docx", 1) _docx_close(dh) end
Example 12: Table indentation
This example shows how to set the table indentation.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh, "Table indentation", "Title") temp = J(1, 5, 1) tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_width(dh, tid1, "pct", 2500) _docx_table_set_ind(dh, tid1, "dxa", 1440) _docx_save(dh, "example12.docx", 1) _docx_close(dh) end
Example 13: Table border
This example shows how to set the table border.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh, "Table border", "Title") temp = J(2, 5, 1) _docx_paragraph_new(dh,"Set the top border to nothing.") tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_border(dh, tid1, "top", "nil") _docx_paragraph_new(dh,"Set the bottom border with pattern") _docx_paragraph_add_text(dh," dashDotStroked and color FF0000.") tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_border(dh, tid1, "bottom", "dashDotStroked", "FF0000") _docx_paragraph_new(dh,"Set the left border with pattern") _docx_paragraph_add_text(dh," dotted and color FF0000.") tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_border(dh, tid1, "start", "dotted", "00FF00") _docx_paragraph_new(dh,"Set the right border to nothing.") tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_border(dh, tid1, "end", "nil") _docx_paragraph_new(dh,"Set the insight horizontal border with pattern") _docx_paragraph_add_text(dh," dotted and color 00FF00.") tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_border(dh, tid1, "insideH", "dotted", "0000FF") _docx_paragraph_new(dh,"Set the insight vertical border with pattern") _docx_paragraph_add_text(dh," dotted and color 0000FF.") tid1 = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_border(dh, tid1, "insideV", "dotted", "0000FF") _docx_save(dh, "example13.docx", 1) _docx_close(dh) end
Example 14: Table cell margin
This example shows how to set the table cell margin.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Table cell margin", "Title") temp = J(3, 4, 1) _docx_paragraph_new(dh,"Table with default cell margin") tid = _docx_add_mata(dh, temp, "%9.0g") _docx_paragraph_new(dh,"Set table cell margin to 240 from top, 120 from") _docx_paragraph_add_text(dh," bottom, 240 from left, and 120 from right.") tid = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_cellmargin(dh, tid, "top", "dxa", 240) _docx_table_set_cellmargin(dh, tid, "bottom", "dxa", 120) _docx_table_set_cellmargin(dh, tid, "left", "dxa", 240) _docx_table_set_cellmargin(dh, tid, "right", "dxa", 120) _docx_save(dh, "example14.docx", 1) _docx_close(dh) end
Example 15: Table cell spacing
This example shows how to set the table cell spacing.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Table cell spacing", "Title") temp = J(3, 4, 1) _docx_paragraph_new(dh,"Set table cell spacing to 120.") tid = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_cellspacing(dh, tid, "dxa", 120) _docx_save(dh, "example15.docx", 1) _docx_close(dh) end
Example 16: Table row alignment
This example shows how to set the table row alignment.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Table row alignment", "Title") temp = J(3, 5, 1) _docx_paragraph_new(dh,"Set the first row to align at left.") _docx_paragraph_add_text(dh," Set the second row to align at center.") _docx_paragraph_add_text(dh," Set the third row to align at right.") tid = _docx_add_mata(dh, temp, "%9.0g") _docx_table_set_width(dh, tid, "pct", 2500) _docx_row_set_halign(dh, tid, 2, "left") _docx_row_set_halign(dh, tid, 2, "center") _docx_row_set_halign(dh, tid, 3, "right") _docx_save(dh, "example16.docx", 1) _docx_close(dh) end
Example 17: Table cell font
This example shows how to set the table cell font property.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Table cell font", "Title") temp = J(1, 5, 1) tid = _docx_add_mata(dh, temp, "%9.0g") _docx_table_mod_cell(dh, tid, 1, 1, "Fonts") _docx_table_mod_cell(dh, tid, 1, 2, "Arial") _docx_cell_set_textfont(dh, tid, 1, 2, "Arial") _docx_table_mod_cell(dh, tid, 1, 3, "Castellar") _docx_cell_set_textfont(dh, tid, 1, 3, "Castellar") _docx_table_mod_cell(dh, tid, 1, 4, "Kunstler Script") _docx_cell_set_textfont(dh, tid, 1, 4, "Kunstler Script") _docx_table_mod_cell(dh, tid, 1, 5, "Wide Latin") _docx_cell_set_textfont(dh, tid, 1, 5, "Wide Latin") _docx_save(dh, "example17.docx", 1) _docx_close(dh) end
Example 18: Table cell text color
This example shows how to set the table cell text color property.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Table cell text color", "Title") temp = J(1, 5, 1) tid = _docx_add_mata(dh, temp, "%9.0g") _docx_table_mod_cell(dh, tid, 1, 1, "Text Colors") _docx_table_mod_cell(dh, tid, 1, 2, "FF0000") _docx_cell_set_textcolor(dh, tid, 1, 2, "FF0000") _docx_table_mod_cell(dh, tid, 1, 3, "00FF00") _docx_cell_set_textcolor(dh, tid, 1, 3, "00FF00") _docx_table_mod_cell(dh, tid, 1, 4, "0000FF") _docx_cell_set_textcolor(dh, tid, 1, 4, "0000FF") _docx_table_mod_cell(dh, tid, 1, 5, "FF00FF") _docx_cell_set_textcolor(dh, tid, 1, 5, "FF00FF") _docx_save(dh, "example18.docx", 1) _docx_close(dh) end
Example 19: Table cell text alignment
This example shows how to set the table cell text alignment property.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Table cell text alignment", "Title") temp = J(1, 4, 1) tid = _docx_add_mata(dh, temp, "%9.0g") _docx_table_mod_cell(dh, tid, 1, 1, "Text Alignment") _docx_table_mod_cell(dh, tid, 1, 2, "left") _docx_cell_set_texthalign(dh, tid, 1, 2, "left") _docx_table_mod_cell(dh, tid, 1, 3, "center") _docx_cell_set_texthalign(dh, tid, 1, 3, "center") _docx_table_mod_cell(dh, tid, 1, 4, "right") _docx_cell_set_texthalign(dh, tid, 1, 4, "right") _docx_save(dh, "example19.docx", 1) _docx_close(dh) end
Example 20: Table cell text vertical alignment
This example shows how to set the table cell text vertical alignment property.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Table cell text vertical alignment", "Title") temp = J(1, 4, 1) tid = _docx_add_mata(dh, temp, "%9.0g") _docx_table_mod_cell(dh, tid, 1, 1, "Test table cell text vertical alignment") _docx_table_mod_cell(dh, tid, 1, 2, " top ") _docx_cell_set_valign(dh, tid, 1, 2, "top") _docx_table_mod_cell(dh, tid, 1, 3, "center") _docx_cell_set_valign(dh, tid, 1, 3, "center") _docx_table_mod_cell(dh, tid, 1, 4, "bottom") _docx_cell_set_valign(dh, tid, 1, 4, "bottom") _docx_save(dh, "example20.docx", 1) _docx_close(dh) end
Example 21: Table cell text size
This example shows how to set the table cell text size property.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Table cell text size", "Title") temp = J(1, 5, 1) tid = _docx_add_mata(dh, temp, "%9.0g") _docx_table_mod_cell(dh, tid, 1, 1, "Text Size") _docx_table_mod_cell(dh, tid, 1, 2, "12") _docx_cell_set_textsize(dh, tid, 1, 2, 12) _docx_table_mod_cell(dh, tid, 1, 3, "18") _docx_cell_set_textsize(dh, tid, 1, 3, 18) _docx_table_mod_cell(dh, tid, 1, 4, "24") _docx_cell_set_textsize(dh, tid, 1, 4, 24) _docx_table_mod_cell(dh, tid, 1, 5, "72") _docx_cell_set_textsize(dh, tid, 1, 5, 72) _docx_save(dh, "example21.docx", 1) _docx_close(dh) end
Example 22: Table cell bold and italic text
This example shows how to set the table cell text to bold or italic.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Set table cell text to bold or italic", "Title") temp = J(1, 3, 1) tid = _docx_add_mata(dh, temp, "%9.0g") _docx_table_mod_cell(dh, tid, 1, 1, "Bold") _docx_cell_set_textbold(dh, tid, 1, 1, 1) _docx_table_mod_cell(dh, tid, 1, 2, "Italic") _docx_cell_set_textitalic(dh, tid, 1, 2, 1) _docx_table_mod_cell(dh, tid, 1, 3, "Bold and Italic") _docx_cell_set_textbold(dh, tid, 1, 3, 1) _docx_cell_set_textitalic(dh, tid, 1, 3, 1) _docx_save(dh, "example22.docx", 1) _docx_close(dh) end
Example 23: Table cell shading
This example shows how to set the table cell shading property.
mata: dh = _docx_new() _docx_paragraph_new_styledtext(dh,"Set table cell shading property", "Title") temp = J(1, 2, 1) tid = _docx_add_mata(dh, temp, "%9.0g") _docx_table_mod_cell(dh, tid, 1, 1, "pct50, fill FF0000, background 0000FF") _docx_cell_set_shading(dh, tid, 1, 1, "FF0000", "0000FF", "pct50") _docx_table_mod_cell(dh, tid, 1, 2, "diagCross, fill 0000FF, background FF0000") _docx_cell_set_shading(dh, tid, 1, 2, "0000FF", "FF0000", "diagCross") _docx_save(dh, "example23.docx", 1) _docx_close(dh) end