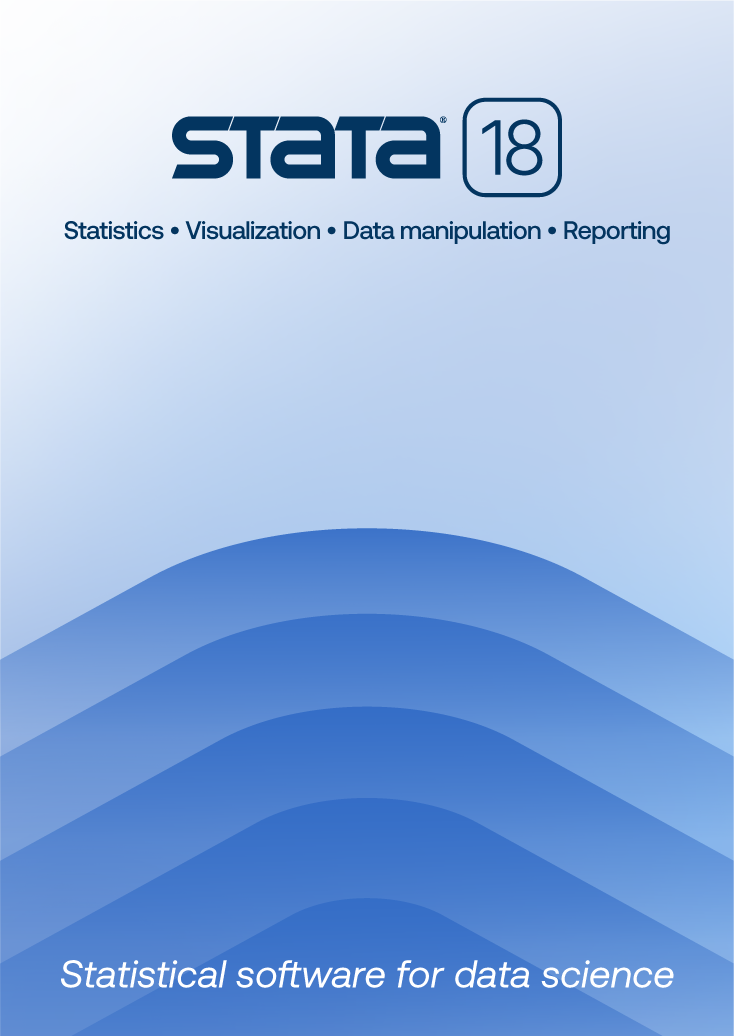
You can write Microsoft Windows applications to interact with Stata via Automation.
The following topics are discussed.
Automation (formerly known as OLE Automation) is a communication mechanism between Microsoft Windows applications. It provides an infrastructure whereby Windows applications (Automation clients) can access and manipulate functions and properties implemented in another application (Automation server). A Stata Automation object exposes internal Stata methods and properties so that Windows programmers can write Automation clients to directly use the services provided by Stata.
In Stata 13, Stata Automation Object is updated to version 1.1 from version 1.0. See details of the changes in secton 3.15.
A Stata Automation object is most useful for situations that require the greatest flexibility to interact with Stata from community-contributed Windows applications. A Stata Automation object enables users to directly access Stata macros, scalars, saved results, and datasets in ways besides the usual log files.
To use a Stata Automation object, the type library has to be registered after Stata is installed on a Microsoft Windows machine.
To register the Stata Automation type library on a Windows non-Vista machine, do the following steps:
To register the Stata Automation type library on Windows Vista, do the following steps:
The following steps will unregister the Stata Automation type library:
To manually register the type library, do the following steps:
For VB.NET or C#:
See http://msdn2.microsoft.com/en-us/library/7314433t(VS.80).aspx for more information.
[VB.NET] dim stataobj As New stata.StataOLEApp()
For VBScript, the user may use the VBScript method CreateObject() to load a Stata Automation object as follows:
[VBScript] dim stataobj set stataobj=CreateObject("stata.StataOLEApp")
Note: Stata Automation is a single-use out-of-process server. This means that creating a new Automation object will launch a new instance of Stata. It is strongly recommended that your software create one Stata Automation object and use it through the execution.
When a Stata Automation object is created, the Stata main window is displayed. Method UtilShowStata() could be used to hide or minimize the Stata main window.
[VB.NET] ' create a Stata Automation object and Stata is launched ' and will be visible. Dim stataobj As New stata.StataOLEApp() ' hide Stata stataobj.UtilShowStata(1) MsgBox("Stata is hidden") ' minimized Stata stataobj.UtilShowStata(2) MsgBox("Stata is minimized") ' restored Stata stataobj.UtilShowStata(0) MsgBox("Stata is restored") stataobj = Nothing
The programmer must release the Stata Automation object to avoid memory leaks.
[VB.NET] stataobj = nothing [VBScript] set stataobj = nothing
The programmer may submit a Stata command from the client to Stata via Automation in two ways. One way uses DoCommand(), and the other uses DoCommandAsync(). DoCommand() returns the return code after the command is finished in Stata and it blocks the client during execution. DoCommandAsync() executes a command asynchronously.
DoCommand() returns the Stata return code from executing the Stata command. A nonzero value means that execution of the command failed.
DoCommand() ignores Stata command exit. The method returns error code 10001 for Stata command program define, while, forvalues, foreach, and input. The programmer who uses Stata Automation needs to pay attention to Stata methods and programs that might block Stata for further user input. Because the Stata window may be hidden or minimized, the end user will not be able to type commands into the Stata Command window. Note: program define, while, forvalues, foreach, and input can still be used inside do- or ado-files.
[VB.NET] dim rc as Integer dim stataobj as new stata.StataOLEApp() rc = stataobj.DoCommand("sysuse auto, clear") if rc = 0 then rc = stataobj.DoCommand("regress mpg price") else ' do error handling end if stataobj = nothing
DoCommandAsync() executes a command asynchronously. It copies the Stata command into a queue and then returns immediately. The command will be executed by Stata when appropriate. The method is equivalent to typing in the Stata Command window. The method has a major advantage over DoCommand(): the user may submit program define, while, forvalues, foreach, and input.
Because the method executes the Stata command asynchronously, the user must be careful about mixing it with DoCommand() and synchronizing the client and server. Sometimes the programmer must make sure that the command submitted via DoCommandAsync() has finished before proceeding to the next operation.
[VB.NET] dim stataobj as new stata.StataOLEApp() ' program define will fail in DoCommand() stataobj.DoCommandAsync("program define myreg") stataobj.DoCommandAsync("regress mpg price") stataobj.DoCommandAsync("end") stataobj.DoCommandAsync("sysuse auto, clear") stataobj.DoCommandAsync("myreg") stataobj = nothing
Here we give an example of determining whether Stata has finished executing a command. The method to use is UtilIsStataFreeEvent(). It monitors the status of Stata and sends an OnFinish event to the client when Stata finishes the execution.
[VB.NET] ' to use WithEvents, stataobj cannot have local scope Public WithEvents stataobj As stata.StataOLEApp Public IsStataFree As Boolean Private Sub testCommandAsync() Dim eN As Integer Dim obs As Integer Dim var As Integer ' create Stata Automation object stataobj = New stata.StataOLEApp() IsStataFree = False obs = 50000 var = 100 stataobj.DoCommandAsync("clear") stataobj.DoCommandAsync("set more off") stataobj.DoCommandAsync("set seed 12345") stataobj.DoCommandAsync("set mem 400M") stataobj.DoCommandAsync("local obs " & obs) stataobj.DoCommandAsync("local var " & var) stataobj.DoCommandAsync("set obs `obs'") stataobj.DoCommandAsync("gen y = 0") stataobj.DoCommandAsync("qui forval i=1/`var' {") stataobj.DoCommandAsync("gen x`i' = runiform()") stataobj.DoCommandAsync("replace y= y+`i'*x`i'") stataobj.DoCommandAsync("}") stataobj.DoCommandAsync("regress y x*") ' make Stata monitor its own status and fire an OnFinish event ' when above commands are finished. The OnFinish event is ' listened and handled by stataobj_OnFinish(). stataobj.UtilIsStataFreeEvent() While IsStataFree = False Application.DoEvents() End While ' Because the value of e(N) depends on the execution of -regress-, ' it is very important to make sure the -regress- has finished. ' Otherwise, eN will not get the correct value. eN = stataobj.StReturnNumeric("e(N)") MsgBox("using DoCommandAsync e(N) = " & eN) stataobj = Nothing End Sub Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click testCommandAsync() End Sub ' handles OnFinish event. Private Sub stataobj_OnFinish(ByVal noCommand As Integer) Handles stataobj.OnFinish IsStataFree = True End Sub
It is useful to have a way to stop Stata when it is executing a long command. There are two ways to do so.
If Stata is visible, the user can stop the execution by clicking the Break button (the button with the big red X) or by holding down Ctrl and pressing the Pause/Break key.
The method UtilSetStataBreak() can also break the execution. Using UtilSetStataBreak() with DoCommand() can be tricky because DoCommand() blocks the client. Hence, the client needs a worker thread to run DoCommand(), and it uses UtilSetStataBreak() from the main thread. On the other hand, using UtilSetStataBreak() with DoCommandAsync() is easy.
Here is an example. DoCommandAsync() sends a long command to Stata. The user could cancel the execution by clicking the cancel button.
[VB.NET] ' the lengthy command sent to Stata is to execute the following do-file, ' test.do ' // test.do ' clear ' set more off ' set seed 12345 ' set mem 200M ' local obs 50000 ' local var 100 ' set obs `obs' ' gen y=0 ' qui forval i=1/`var' { ' gen x`i' = runiform() ' replace y= y+`i'*x`i' ' } ' regress y x* ' ' //end of test.do ' ' to use WithEvents, stataobj cannot have local scope Public WithEvents stataobj As stata.StataOLEApp Public IsStataFree As Boolean Private Sub testCommandAsync() ' create Stata Automation object stataobj = New stata.StataOLEApp() stataobj.DoCommandAsync("do test.do") ' make Stata monitor its own status and fire an OnFinish event ' when above commands are finished. stataobj.UtilIsStataFreeEvent() While IsStataFree = False Application.DoEvents() End While stataobj = Nothing End Sub Private Sub butCancel_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles butCancel.Click If stataobj Is Nothing Then ' do nothing Else stataobj.UtilSetStataBreak() End If End Sub
Method MacroValue() gets the value of a macro from Stata. The macro value is returned as a string. If the macro with the specified name cannot be found, the method returns an empty string.
The name of a local macro needs to be prefixed with “_” when it is submitted via MacroValue(), because Stata automatically prefixes local macros with “_”.
[VB.NET] Imports System ' get macro Dim stataobj As New stata.StataOLEApp() Dim mGlobal, mLocal As String stataobj.DoCommand("global mGlobal = ""test global macro""") stataobj.DoCommand("local mLocal = ""test local macro""") ' get global macro mGlobal mGlobal = stataobj.MacroValue("mGlobal") ' get local macro mLocal. Note: local macro name need have a "_" prefix. mLocal = stataobj.MacroValue("_mLocal") Console.WriteLine("global macro mGlobal : " & mGlobal & ";" & "local > macro mLocal : " & mLocal & vbCrLf) ' if the macro name is wrong, MacroValue returns empty string. mLocal = stataobj.MacroValue("mLocal") Console.WriteLine("local macro mLocal with wrong name(returns empty > string): " & mLocal) stataobj = Nothing
Methods ScalarNumeric() and ScalarString() get the value of a scalar from Stata. Method ScalarType() determines whether the scalar is numeric or string.
[VB.NET] Imports System ' get scalar Dim stataobj As New stata.StataOLEApp() stataobj.DoCommand("scalar na=10") stataobj.DoCommand("scalar sa=""this is a test""") Dim na As Double Dim sa As String Dim type As Integer ' ' type = 0 : the scalar with the name is not found. ' 1 : the scalar with the name is numeric. ' 2 : the scalar with the name is string. ' type = stataobj.ScalarType("na") If type = 1 Then Console.WriteLine("scalar na is of numeric type") End If na = stataobj.ScalarNumeric("na") ' na is 10 now sa = stataobj.ScalarString("sa") ' sa is "this is a test" now Console.WriteLine("numeric na=" & na & "; string sa=" & sa) ' get the scalar with wrong data type sa = stataobj.ScalarNumeric("sa") ' sa is Stata missing value now na = stataobj.ScalarString("na") ' na is 0 now, because it has the ' double value casted from an ' empty string. Console.WriteLine("wrong type:numeric na=" & na & ";string sa=" & sa) ' test for nonexistent scalars na = stataobj.ScalarNumeric("not_exist") ' na is misval sa = stataobj.ScalarString("not_exist") ' sa is empty string Console.WriteLine("not exist: numeric na=" & na & ";string sa=" & sa) stataobj = Nothing
Methods StReturnNumeric() and StReturnString() get the value of a saved result from Stata. Method StReturnType() determines whether the saved result is numeric or string. Section 3.11 discusses the saved result matrix.
[VB.NET] Imports System Dim stataobj As New stata.StataOLEApp() ' get saved return and type ' Types of return: ' 15 - numeric ' 3059 - string Dim c_current_date As String Dim c_current_date_type As Integer Dim c_max_matsize As Integer Dim c_max_matsize_type As Integer Dim c_maxdouble As Double Dim c_maxdouble_type As Integer c_current_date = stataobj.StReturnString("c(current_date)") c_current_date_type = stataobj.StReturnType("c(current_date)") c_max_matsize = stataobj.StReturnNumeric("c(max_matsize)") c_max_matsize_type = stataobj.StReturnType("c(max_matsize)") c_maxdouble = stataobj.StReturnNumeric("c(maxdouble)") c_maxdouble_type = stataobj.StReturnType("c(maxdouble)") Console.WriteLine("current_date type:" &c_current_date_type & ";" ) Console.WriteLine("current_date:" & c_current_date & vbCrLf) Console.WriteLine("max_matsize type:" &c_max_matsize_type & ";" ) Console.WriteLine("max_matsize:" & c_max_matsize & vbCrLf) Console.WriteLine("maxdouble type:" & c_maxdouble_type & ";") Console.WriteLine("max_double:" & c_maxdouble & vbCrLf) ' get e return Dim e_N As Integer Dim e_N_type As Integer Dim e_F As Double Dim e_F_type As Integer Dim e_depvar As String Dim e_depvar_type As Integer stataobj.DoCommand("sysuse auto, clear") stataobj.DoCommand("regress mpg price") e_N = stataobj.StReturnNumeric("e(N)") e_N_type = stataobj.StReturnType("e(N)") e_F = stataobj.StReturnNumeric("e(F)") e_F_type = stataobj.StReturnType("e(F)") e_depvar = stataobj.StReturnString("e(depvar)") e_depvar_type = stataobj.StReturnType("e(depvar)") Console.WriteLine("e(N) type:" & e_N_type & ";") Console.WriteLine("e(N):" & e_N & vbCrLf) Console.WriteLine("e(F) type:" & e_F_type & ";") Console.WriteLine("e(F):" & e_F & vbCrLf) Console.WriteLine("e(depvar) type:" & e_depvar_type & ";") Console.WriteLine("e(depvar):" & e_depvar & vbCrLf) ' get r return Dim r_N As Integer Dim r_N_type As Integer Dim r_mean As Double Dim r_mean_type As Integer stataobj.DoCommand("sysuse auto, clear") stataobj.DoCommand("summarize") r_N = stataobj.StReturnNumeric("r(N)") r_N_type = stataobj.StReturnType("r(N)") r_mean = stataobj.StReturnNumeric("r(mean)") r_mean_type = stataobj.StReturnType("r(mean)") Console.WriteLine("r(N) type:" & r_N_type & ";") Console.WriteLine("r(N):" & r_N & vbCrLf) Console.WriteLine("r(mean) type:" & r_mean_type & ";") Console.WriteLine("r(mean):" & r_mean & vbCrLf) stataobj = Nothing
Stata system missing value “.” is represented by an arbitrarily large positive value. Any number greater than or equal to this value will be treated as a missing value in Stata. There are two ways to determine whether a number will be treated as a missing value.
Method UtilIsStMissingValue(double valueToCheck) returns True if the valueToCheck is greater than or equal to the Stata system missing value.
Programmers can also use the method UtilGetStMissingValue() to get a local copy of the Stata system missing value and then compare numbers with it in the client side. This way saves future round trips of sending data to the Stata server.
[VB.NET] Imports System Dim stataobj As New stata.StataOLEApp() Dim misVal, misVal1, misVal2 As Double Dim isMissing As Boolean misVal = stataobj.UtilGetStMissingValue() isMissing = stataobj.UtilIsStMissingValue(misVal) If isMissing = True Then ' is True Console.WriteLine(misVal & " is treated as missing." & vbCrLf) End If misVal1 = 5.0E+307 isMissing = stataobj.UtilIsStMissingValue(misVal1) If isMissing = False Then ' is false Console.WriteLine(misVal1 & " is not treated as missing." & vbCrLf) End If misVal2 = 2 * misVal1 isMissing = stataobj.UtilIsStMissingValue(misVal2) If isMissing = True Then ' is True Console.WriteLine(misVal2 & " is treated as missing." & vbCrLf) End If stataobj = Nothing
Method MatrixData() returns a Stata matrix as a one-dimensional double array. The size of the array is the number of entries in the matrix. The method returns the full matrix even for a symmetric matrix.
Methods MatrixRowDim() and MatrixColDim() return the number of rows and the number of columns of the matrix, respectively. Methods MatrixRowNames() and MatrixColNames() return the row names and the column names of the matrix in a string array.
[VB.NET] Imports System ' get matrix Dim stataobj As New stata.StataOLEApp() Dim eV As Double() Dim i, j, t As Integer Dim row, column As Integer Dim rowNames, columnNames As String() stataobj.DoCommand("sysuse auto, clear") stataobj.DoCommand("regress mpg price") ' get number of rows of e(V) row = stataobj.MatrixRowDim("e(V)") ' get row names of matrix e(V) rowNames = stataobj.MatrixRowNames("e(V)") ' get number of columns of e(V) column = stataobj.MatrixColDim("e(V)") ' get column names of matrix e(V) columnNames = stataobj.MatrixColNames("e(V)") ' get matrix eV = stataobj.MatrixData("e(V)") ' display matrix Console.WriteLine("matrix e(V)[" & row & ", " & column & "]" & vbCrLf) ' display column header Console.WriteLine(String.Format("{0,20}", "")) For i = 0 To column - 1 Console.WriteLine(String.Format("{0,20}", columnNames(i))) Next Console.WriteLine(vbCrLf) For i = 0 To row - 1 ' display row header Console.WriteLine(String.Format("{0,-20}", rowNames(i))) t = i * column For j = 0 To column - 1 Console.WriteLine(String.Format("{0,20}", eV(t).ToString("e8"))) t = t + 1 Next Console.WriteLine(vbCrLf) Next MsgBox("Got matrix information for e(V)") stataobj = Nothing
Method StVariableIndex() returns the index of the variable given the name. If the variable with the name is not found, StVariableIndex() returns −111.
Method StVariableName() returns the name of the variable given the index. A valid variable index is greater than or equal to 1 and is less than or equal to the number of variables. If the variable index is not valid, StVariableName() returns an empty string. Method VariableNameArray() returns a string array of all variable names.
[VB.NET] Imports System ' get variable index Dim stataobj As New stata.StataOLEApp() Dim varIndex As Integer stataobj.DoCommand("sysuse auto, clear") ' get the variable index of make varIndex = stataobj.StVariableIndex("make") Console.WriteLine("make-variable index:" & varIndex & vbCrLf) ' if the variable cannot be found varIndex = stataobj.StVariableIndex("not_exist") Console.WriteLine("not_exist-variable index:" & varIndex & vbCrLf) ' get variable name Dim varName As String ' get the first variable name varName = stataobj.StVariableName(1) Console.WriteLine("var 1 name:" & varName & vbCrLf) ' get the last variable name varName = stataobj.StVariableName(12) Console.WriteLine("var 12 name:" & varName & vbCrLf) ' try an out-of-range variable index varName = stataobj.VariableLabel("13") Console.WriteLine("var 13 (out of range) name:" & varName & vbCrLf) ' get all variable names in an array Dim varNames As String() Dim i As Integer ' get all variable names varNames = stataobj.VariableNameArray() For i = 0 To varNames.Length - 1 Console.WriteLine("var " & i + 1 & " name:" & varNames(i) & vbCrLf) Next stataobj = Nothing
Method VariableType() returns an integer that represents the data type of the variable. Possible data type values include the following:
0: type not defined 1-244: string variable 251: byte 252: integer 253: long integer 254: float 255: double
The result can be compared with the values in stata.StataVariableTypes enum type.
Method VariableTypeArray() returns a data type array for all variables.
[VB.NET] Imports System ' get variable data type Dim stataobj As New stata.StataOLEApp() Dim varType As Byte stataobj.DoCommand("sysuse auto, clear") ' get the type of make varType = stataobj.VariableType("make") ' vartype for make is 18. Test against stata.StataVariableTypes If varType = stata.StataVariableTypes.vstUndef Then Console.WriteLine("data type undefined") End If If varType <= stata.StataVariableTypes.vstString And varType >= 1 Then Console.WriteLine("data type string.") End If If varType = stata.StataVariableTypes.vstByte Then Console.WriteLine("data type byte") End If If varType = stata.StataVariableTypes.vstInt Then Console.WriteLine("data type integer") End If If varType = stata.StataVariableTypes.vstLong Then Console.WriteLine("data type long") End If If varType = stata.StataVariableTypes.vstFloat Then Console.WriteLine("data type float") End If If varType = stata.StataVariableTypes.vstDouble Then Console.WriteLine("data type double") End If Console.WriteLine(vbCrLf) ' get the type of price varType = stataobj.VariableType("price") ' vartype for price is 252, which corresponds to integer. Console.WriteLine("var price-data type:" & varType & vbCrLf) ' vartype is 0 if it cannot be found. varType = stataobj.VariableType("not_exist") Console.WriteLine("var not_exist-data type:" & varType & vbCrLf) ' get data type array for all variables Dim varTypes As Byte() Dim i As Integer ' get the types of all variables varTypes = stataobj.VariableTypeArray() For i = 0 To varTypes.Length - 1 Console.WriteLine("var " &i+1&"-data type:" & varTypes(i) & vbCrLf) Next stataobj = Nothing
Method VariableLabel() returns the label of a variable. Method VariableLabelArray() returns labels for all variables.
[VB.NET] Dim stataobj As New stata.StataOLEApp() Dim varLabel As String stataobj.DoCommand("sysuse auto, clear") ' get the variable label of make varLabel = stataobj.VariableLabel("make") Console.WriteLine("var make-label:" & varLabel & vbCrLf) ' test for nonexistent variables varLabel = stataobj.VariableLabel("not_exist") Console.WriteLine("var not_exist-label:" & varLabel & vbCrLf) ' get variable labels for all variables Dim varLabels As String() Dim i As Integer ' get all variable labels varLabels = stataobj.VariableLabelArray() For i = 0 To varLabels.Length - 1 Console.WriteLine("var " &i+1& "-label:" & varLabels(i) & vbCrLf) Next stataobj = Nothing
Method VariableValLabel() returns the value label for a variable. It returns the empty string if the variable does not have a value label.
Method VariableValLabelArray() returns a one-dimensional string array for all value labels for all variables.
A value label contains several value–label pairs. Method VariableValLabelCount() returns the number of value–label pairs in a value label. Method VariableValLabelValueArray() returns an integer array of all values in a value label. Method VariableValLabelLabelArray() returns a string array of all labels in a value label.
[VB.NET] Imports System ' test value labels Dim stataobj As New stata.StataOLEApp() Dim valLabelName As String Dim varIndex As Integer stataobj.DoCommand("sysuse auto, clear") ' get the value label of variable foreign varIndex = stataobj.StVariableIndex("foreign") If varIndex > 0 Then ' get the variable value label valLabelName = stataobj.VariableValLabel(varIndex) ' valLabelName is -origin- Console.WriteLine("var foreign-value label:"&valLabelName& vbCrLf) End If ' get the value label of variable make varIndex = stataobj.StVariableIndex("make") If varIndex > 0 Then ' get the variable value label valLabelName = stataobj.VariableValLabel(varIndex) ' valLabelName is empty string Console.WriteLine("var make-value label:" & valLabelName & vbCrLf) End If Dim valLabelNames As String() Dim i As Integer ' get the value labels valLabelNames = stataobj.VariableValLabelArray() For i = 0 To valLabelNames.Length - 1 Console.WriteLine("value label-"&i+1& &valLabelNames(i)& vbCrLf) Next ' value label origin has two values, 0 and 1, and one label for each value. ' get the number of values in a value label Dim valCount As Integer valCount = stataobj.VariableValLabelCount("origin") Console.WriteLine("value label origin has " &valCount& " values"&vbCrLf) ' get each label Dim valLabel As String For i = 0 To valCount - 1 valLabel = stataobj.VariableValLabelForValue("origin", i) Console.WriteLine("origin "&i+1& "-th label is: "&valLabel& vbCrLf) Next ' get all values in an array and all labels in another array Dim values As Integer() Dim labels As String() ' get all values in value label origin values = stataobj.VariableValLabelValueArray("origin") ' get all labels in value label origin labels = stataobj.VariableValLabelLabelArray("origin") For i = 0 To values.Length - 1 Console.WriteLine("origin " &i+1& "-th value is: "&values(i)&vbCrLf) Console.WriteLine("origin " &i+1& "-th label is: "&values(i)&vbCrLf) Next stataobj = Nothing
Methods VariableData() and VariableDataFromName() return an observation array for a variable. The returned array contains variants. The array can be either string or double. Programmers need to determine the data type and make the correct type cast.
Both methods take the start observation index, startObs, and end observation index, endObs, as the second and the third arguments. Let nobs be the number of observations in the dataset. The following relations must hold for inputs:
Otherwise, both methods produce an ERROR_INVALID_PARAMETER error and return a NULL array.
[VB.NET] Imports System ' get data from one variable Dim stataobj As New stata.StataOLEApp() Dim varName As String Dim varType As Integer Dim nobs As Integer Dim i As Integer stataobj.DoCommand("sysuse auto, clear") ' get the type and data of the first variable - make varName = stataobj.StVariableName(1) varType = stataobj.VariableType(varName) ' get number of observations stataobj.DoCommand("scalar nobs=_N") nobs = stataobj.ScalarNumeric("nobs") If varType = stata.StataVariableTypes.vstUndef Then ' undefined data type - do error handling Exit Sub End If If varType <= stata.StataVariableTypes.vstString And varType >= 1 Then ' string variable Dim varData As String() ' get all observations varData = stataobj.VariableData(1, 1, nobs) For i = 0 To nobs - 1 Console.WriteLine(i + 1 & ":" & varData(i) & vbCrLf) Next End If ' get the type and data of variable price varType = stataobj.VariableType("price") ' get number of observations stataobj.DoCommand("scalar nobs=_N") nobs = stataobj.ScalarNumeric("nobs") If varType = stata.StataVariableTypes.vstUndef Then ' undefined data type - do error handling Exit Sub End If If varType > stata.StataVariableTypes.vstString Then ' numeric variable Dim varData As Double() ' get all observations varData = stataobj.VariableDataFromName("price", 1, nobs) For i = 0 To nobs - 1 Console.WriteLine(i + 1 & ":" & varData(i) & vbCrLf) Next End If stataobj = Nothing
Method VariablesData() returns a variant array of observations of several variables at once. The arguments of the method are
They must satisfy the following conditions:
Otherwise, the method produces an INVALID_PARAMETER error and returns a NULL array. The method returns observations for all variables with index from startVariableIndex to endVariableIndex.
Because the variables may be both string and numeric, a variant array must be used instead of a regular two-dimensional array. To get the data for each variable, the programmer must do the following steps:
' declare dataArr as a variant array dim dataArr() as object dim varType as integer dim i as integer ' get observations of variable 1 and 2 dataArr = stataobj.VariablesData(1, 2, 1, 20) dim dataString as String() dim dataDbl as double() 'find the data type of the first variable varType = stataobj.VariableType(1) ' suppose it is a string variable, assign the first element ' dataArr to a string array dataString = dataArr(0) ' if it is numeric, assign it to dataDbl ' then you may access each observation of the first variable through ' elements of dataString for i=0 to dataString.length-1 Console.WriteLine(dataString(i)) next
Here is an example.
[VB.NET] Imports System ' get data of several variables at once Dim stataobj As New stata.StataOLEApp() Dim varName As String Dim varType As Integer Dim nobs As Integer Dim nvar As Integer Dim i As Integer Dim j As Integer stataobj.DoCommand("set more off") stataobj.DoCommand("sysuse auto, clear") ' get number of observations and number of vars stataobj.DoCommand("describe") nobs = stataobj.StReturnNumeric("r(N)") nvar = stataobj.StReturnNumeric("r(k)") ' ' get data of variables from 1 to 3. Because there are both ' string and numeric variables, we need to use the variant ' array. ' Dim varStart, varEnd As Integer Dim dataArr() As Object ' get data from variables from 1 to 3 varStart = 1 varEnd = 3 dataArr = stataobj.VariablesData(1, 3, 1, nobs) ' display For i = varStart To varEnd ' display var name varName = stataobj.StVariableName(i) Console.WriteLine(varName & vbCrLf) ' get varType varType = stataobj.VariableType(varName) If varType <= stata.StataVariableTypes.vstString And varType >= 1 > Then ' string type Dim dataString As String() dataString = dataArr(i - varStart) ' display all data For j = 0 To nobs - 1 Console.WriteLine(j + 1 & ":" & dataString(j) & vbCrLf) Next Console.WriteLine(vbCrLf) End If If varType > stata.StataVariableTypes.vstString Then ' numeric type Dim dataDbl As Double() dataDbl = dataArr(i - varStart) ' display all data For j = 0 To nobs - 1 Console.WriteLine(j + 1 & ":" & dataDbl(j) & vbCrLf) Next Console.WriteLine(vbCrLf) End If Next stataobj = Nothing
According to Microsoft:
"The VBSCRIPT active scripting engine supplied by Microsoft only supports the indexing of SAFEARRAYs of VARIANTs. While VBSCRIPT is capable of accepting arrays of non-variant type for the purposes of boundary checking and passing it to other Automation objects, the engine does not allow manipulation of the array contents at this time."
Hence, if a script attempts to reference the elements of an array returned by a method such as MatrixRowNames(), the script engine reports: "Object doesn't support this property or method stata.MatrixRowNames()".
In order to solve this problem, the following methods are provided for programming in VBScript. The method for VBScript returns a variant array instead of returning an array of specific data type like the corresponding regular method does.
VBScript | VB.NET |
---|---|
MatrixDataAsVariants() | MatrixData() |
MatrixRowNamesAsVariants() | MatrixRowNames() |
MatrixColNamesAsVariants() | MatrixColNames() |
VariableDataAsVariants() | VariableData() |
VariableDataFromNameAsVariants() | VariableDataFromName() |
VariablesDataAsVariants() | VariablesData() |
VariablesAllDataAsVariants() | VariablesAllData() |
VariableLabelAsVariants() | VariableLabelArray() |
VariableNameAsVariants() | VariableNameArray() |
VariableTypeAsVariants() | VariableTypeArray() |
VariableValLabelAsVariants() | VariableValLabelArray() |
VariableValLabelLabelAsVariants() | VariableValLabelLabelArray() |
VariableValLabelValueAsVariants() | VariableValLabelValueArray() |
Here is an example.
[VBScript] ' VBScript sample code dim stataobj set stataobj=CreateObject("stata.StataOLEApp") stataobj.DoCommand("sysuse auto") stataobj.DoCommand("regress mpg price") ' get variable names as an array of variants dim varNames dim i varNames = stataobj.VariableNameAsVariants() For i=0 to 5 msgbox "var " & i+1 & " " & varNames(i) Next ' get variable labels as an array of variants dim varLabels varLabels = stataobj.VariableLabelAsVariants() msgbox "1st variable label =" & CStr(varLabels(0)) ' get the matrix column names as an array of variants dim colNames colNames = stataobj.MatrixRowNamesAsVariants("e(V)") For i=0 to 1 msgbox "eV's " & i & "-th column name: " & CStr(colNames(i)) Next ' get matrix data as an array of variants. dim matentry matentry = stataobj.MatrixDataAsVariants("e(V)") For i=0 to 3 msgbox "eV's " & i & "-th = : " & matentry(i) Next ' get value label -origin-'s values as an array of variants dim values dim valcount valcount = stataobj.VariableValLabelCount("origin") values = stataobj.VariableValLabelValueAsVariants("origin") For i=0 to valcount-1 msgbox "Value label origin's " & i & "-th value=" & CInt(values(i)) Next ' get data as an array of variants dim make dim price make = stataobj.VariableDataAsVariants(1, 1, 5) price = stataobj.VariableDataAsVariants(2, 1, 5) For i=0 to 4 msgbox i+1 & "-th observation of variable make=" & make(i) msgbox i+1 & "-th observation of variable price=" & price(i) Next set stataobj=nothing
In Stata 12, string types are
String storage Maximum type length Bytes ---------------------------------- str1 1 1 str2 2 2 ... . . ... . . ... . . str244 244 244 ----------------------------------
In Stata 13, string types become
String storage Maximum type length Bytes ----------------------------------------- str1 1 1 str2 2 2 ... . . ... . . ... . . str2045 2045 2045 strL 2000000000 2000000000 -----------------------------------------
Stata Automation Object in Stata 13 is updated to version 1.1 to work with the new string data types.
In version 1.0,
vstUndef: type not defined 1-vstString: string variable with maximum length of the value vstByte: byte vstInt: integer vstLong: long integer vstFloat: float vstDouble: double
where vstString is 244.
In version 1.1,
1-(vstString-1): string variable with maximum length of the value All string types str#, where #>=vstString, and strL are mapped to vstString for backward compatibility. Hence, you can no longer rely on the return value of VariableType() to determine the maximum width of the string variable.
VariableType(BSTR variableName,BYTE *variableType) VariableTypeArray(BSTR variableName,BYTE *variableType)
For all string types, str#, where #>=vstString, and strL are mapped to vstString for backward compatibility.
The following code still works:
If varType > stata.StataVariableTypes.vstString Then ' numeric type Else ' String type End If
But you can no longer rely on the return value of VariableType() to determine the maximum width of the string variable.
The same changes apply to VariableTypeArray() and VariableTypeAsVariants().
VariableData(LONG varNo, LONG obsStart, LONG obsEnd, VARIANT *data) VariableDataAsVariants(LONG varNo, LONG obsStart, LONG obsEnd, VARIANT *data) VariableDataFromName(BSTR variableName, ULONG obsStart, ULONG obsEnd, VARIANT *data) VariableDataFromNameAsVariants(BSTR variableName, ULONG obsStart, ULONG obsEnd, VARIANT *data) VariablesData(ULONG vStart, ULONG vEnd, ULONG oStart, ULONG oEnd, VARIANT *data) VariablesDataAsVariants(ULONG vStart, ULONG vEnd, ULONG oStart, ULONG oEnd, VARIANT *data) VariablesAllData(ULONG oStart, ULONG oEnd, VARIANT *data) VariablesAllDataAsVariants(ULONG oStart, ULONG oEnd, VARIANT *data)
If the string variable type is str#, the function returns the full contents of the variable in VARIANT *data. If the string variable type is strL, the function returns the content up to 2,045 bytes or to the first binary 0, whichever is less, of each observation in the VARIANT *data.
ScalarString(BSTR scalarName, BSTR *scalarValue)
The function returns the string scalar content up to 2,045 bytes or to the first binary 0, whichever is less.
VariableDataStrLFromName(BSTR variableName, LONG obs, VARIANT *data) VariableDataStrL(LONG varNo, LONG obs, VARIANT *data) return strL data as a byte array in VARIANT *data VariableIsStrLFromName(BSTR variableName, BYTE *isStrL) VariableIsStrL(ULONG var, BYTE *isStrL) 0 : not strL 1 : strL ScalarByteArray(BSTR scalarName, VARIANT *data) return strL data as a byte array in VARIANT *data
The existing code will work for both version 1.0 and 1.1 if there are no assumptions or usage in the code that 244 is the maximum length of a string variable. For instance, all examples in the document work for both version 1.0 and 1.1.
If your code needs to be run on both version 1.0 and version 1.1, you may
Dim cV As Integer cV = stataobj.StReturnNumeric("c(stata_version)") If cV >= 13 Then ' verion 1.1, new functions are supported Else ' version 1.0, new functions are not supported End If
strL.dta dataset contains one variable content, which has two observations. The first observation is an ASCII text string. The second observation is a binary GIF file.
[VB.NET] stataobj.DoCommand("use mp.dta, clear") Dim BytStr() As Byte ' Get the first observation as a byte array BytStr = stataobj.VariableDataStrLFromName("content", 1) Dim BytBinary() As Byte ' Get the first observation as a byte array BytBinary = stataobj.VariableDataStrLFromName("content", 2) Dim Str As String ' Convert the first observation to a String Str = System.Text.Encoding.ASCII.GetString(BytStr) ' Display the String in a RichTextBox RtbMP.Text = Str ' Convert the second observation to a IO Stream Dim BinStr As System.IO.Stream = New System.IO.MemoryStream(BytBinary) ' Display the image in a PictureBox PicbMP.Image = Image.FromStream(BinStr)
For VBScript, you can use ADODB.Stream to work with the binary data.
[VBScript] dim stataobj set stataobj=CreateObject("stata.StataOLEApp") stataobj.DoCommand("use mp.dta, clear") Dim BytBinary BytBinary = stataobj.VariableDataStrLFromName("content", 2) Dim BinStr Set BinStr = CreateObject("ADODB.Stream") ' Specify stream type to binary BinStr.Type = 1 ' Write binary data BinStr.Open BinStr.Write byt ' Save stream data to file BinStr.SaveToFile "mp.gif", 2
In Stata 14, the maximum number of observations is increased from 2,147,483,647 to 281,474,976,710,655 in 64-bit Stata/MP.
The number 281,474,976,710,655 is beyond the range of ULONG 0-4,294,967,295. The currently supported methods like VariableData() are not able to access the observations beyond 4,294,967,295. To deal with this, Stata OLE Automation Object is updated to version 1.2 and the following new methods are added:
VarDataBigObs(LONG variableIndex, DOUBLE startObservation, DOUBLE endObservation, VARIANT *data) VarDataBigObsAsVariants(LONG variableIndex, DOUBLE startObservation, DOUBLE endObservation, VARIANT *data) VarDataBigObsFromName(BSTR variableName, DOUBLE startObservation, DOUBLE endObservation, VARIANT *data) VarDataBigObsFromNameAsVariants(BSTR variableName, DOUBLE startObservation, DOUBLE endObservation, VARIANT *data) VarsDataBigObs(ULONG startVariableIndex, ULONG endVariableIndex, DOUBLE startObservation, DOUBLE endObservation, VARIANT *data) VarsDataBigObsAsVariants(ULONG startVariableIndex, ULONG endVariableIndex, DOUBLE startObservation, DOUBLE endObservation, VARIANT *data) VarsAllDataBigObs(DOUBLE startObservation, DOUBLE endObservation, VARIANT *data) VarsAllDataBigObsAsVariants(DOUBLE startObservation, DOUBLE endObservation, VARIANT *data) VarDataBigObsStrL([in]ULONG var, DOUBLE obs, VARIANT *var) VarDataBigObsStrLFromName(BSTR strVariableName, DOUBLE obs, VARIANT *var)
Note that startObservation, endObservation, and obs are doubles that allow you to specify integers beyond 4,294,967,295. They will be cast to integers in Stata; hence, 5.7 becomes 5.
Also note that the maximum size of array is 4,294,967,295; hence, it may not be possible to return all observations from a variable in a single method call even if there is plenty of memory.
Method: |
DoCommand(BSTR stataCommand, LONG* stataErrorCode) |
---|---|
Description: |
Execute a Stata command. |
Input: |
stataCommand: a string of Stata command. |
Output: |
stataErrorCode: value of the Stata return code from executing the Stata command. A nonzero return code means the execution of the command failed. |
Return: |
S_OK: all is well. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
|
Method: |
DoCommandAsync(BSTR stataCommand, LONG* stataErrorCode) |
---|---|
Description: |
Execute a Stata command asynchronously. (The method copies the Stata command into a queue and then returns immediately. The command will be executed by Stata when it is its turn.) |
Input: |
stataCommand: a string of the Stata command. |
Output: |
stataErrorCode: the return code is always zero because the command is only copied into the queue. |
Return: |
S_OK: all is well. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
Because the method executes the Stata command asynchronously, knowing whether a Stata command has finished before starting the next operation is sometimes important. The programmer could use the UtilIsStataFreeEvent() method to check whether a command has finished execution. See http://www.stata.com/automation/, section 3.7, for details. |
Method: |
MacroValue(BSTR macroName, BSTR *macroValue) |
---|---|
Description: |
Get the value as a BSTR for a Stata macro with macroName. |
Input: |
macroName: a BSTR of the macro name. |
Output: |
macroValue: the value of the macro as a string. |
Return: |
S_OK: all is well. ERROR_OUTOFMEMORY: system out of memory. |
Note: | The name of local macro must be prefixed by “_”; i.e., to get the value of macro defined in Stata by local lm="test", the programmer needs to call the method as value=stataobj.MacroValue("_lm"). |
Method: |
ScalarType(BSTR scalarName, LONG *scalarType) |
---|---|
Description: |
Determine whether the scalar is numeric or string. |
Input: |
scalarName: scalar name as a BSTR. |
Output: |
scalarType: type of the scalar as an integer. Possible values are 0: not found |
Return: |
S_OK |
Note: |
The length of the scalar name must be less than 32; otherwise, the name will be truncated. |
Method: |
ScalarNumeric(BSTR scalarName, Double *scalarValue) |
---|---|
Description: |
Get the value of a numerical scalar. |
Input: |
scalarName: the scalar name. |
Output: |
scalarValue: the value of the scalar. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: cannot be found or is a string scalar. E_FAIL: Stata error when trying to load the scalar from memory. |
Note: |
|
Method: |
ScalarString(BSTR scalarName, BSTR *scalarValue) |
---|---|
Description: |
Get the value of a string scalar. |
Input: |
scalarName: the scalar name. |
Output: |
scalarValue: the string value of the scalar. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: cannot be found or is a numeric scalar. E_FAIL: Stata error when trying to load the scalar. |
Note: |
|
Method: |
StReturnType(BSTR returnName, LONG *returnType) |
---|---|
Description: |
Determine the type of a Stata saved result. |
Input: |
returnName: the name of the saved result. |
Output: |
returnType: the return type. Possible values are 1: numeric return |
Return: |
S_OK: all is well. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
|
Method: |
StReturnNumeric(BSTR returnName, DOUBLE *returnValue) |
---|---|
Description: |
Get the value of a numerical return. |
Input: |
returnName: the return name. |
Output: |
returnValue: the return value. |
Return: |
S_OK: all is well. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
|
Method: |
StReturnString(BSTR returnName, BSTR *returnString) |
---|---|
Description: |
Get the value of a string return. |
Input: |
returnName: the return name. |
Output: |
returnString: string value of the return. |
Return: |
S_OK: all is well. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
|
Method: |
MatrixData(BSTR matrixName, VARIANT *matrix) |
---|---|
Description: |
Get the data of a matrix. |
Input: |
matrixName: the matrix name. |
Output: |
matrix: a one-dimensional double array of the matrix entries. |
Return: |
S_OK: all is well. E_FAIL: failed. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
|
Method: |
MatrixDataAsVariants(BSTR matrixName, VARIANT *matrix) |
---|---|
Description: |
Get the data of a matrix. |
Input: |
matrixName: the matrix name. |
Output: |
matrix: a one-dimensional variant array of the matrix entries. |
Return: |
S_OK: all is well. E_FAIL: failed. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
|
Method: |
MatrixRowNames(BSTR matrixName, VARIANT *matrixRowNames) |
---|---|
Description: |
Get the row names of a matrix. |
Input: |
matrixName: the matrix name. |
Output: |
matrixRowNames: a string array of the matrix row names. |
Return: |
S_OK: all is well. E_FAIL: failed. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The maximum length of the matrix name is 32. |
Method: |
MatrixRowNamesAsVariants(BSTR matrixName, VARIANT *matrixRowNames) |
---|---|
Description: |
Get the row names of a matrix. |
Input: |
matrixName: the matrix name. |
Output: |
matrixRowNames: a variant array of the matrix row names. |
Return: |
S_OK: all is well. E_FAIL: failed. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The method can be used in VBScript. |
Method: |
MatrixColNames(BSTR matrixName, VARIANT *matrixColNames) |
---|---|
Description: |
Get the column names of a matrix. |
Input: |
matrixName: the matrix name. |
Output: |
matrixColNames: a string array of the matrix column names. |
Return: |
S_OK: all is well. E_FAIL: failed. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The maximum length of the matrix name is 32. |
Method: |
MatrixColNamesAsVariants(BSTR matrixName, VARIANT *matrixColNames) |
---|---|
Description: |
Get the column names of a matrix. |
Input: |
matrixName: the matrix name. |
Output: |
matrixColNames: a variant array of the matrix column names. |
Return: |
S_OK: all is well. E_FAIL: failed. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The method can be used in VBScript. |
Method: |
MatrixRowDim(BSTR matrixName, LONG *matrixRowNo) |
---|---|
Description: |
Get the number of rows of a matrix. |
Input: |
matrixName: the matrix name. |
Output: |
matrixRowNo: number of rows of the matrix. |
Return: |
S_OK: all is well. E_FAIL: failed. |
Note: |
|
Method: |
MatrixColDim(BSTR matrixName, LONG *matrixColNo) |
---|---|
Description: |
Get the number of columns of a matrix. |
Input: |
matrixName: the matrix name. |
Output: |
matrixColNo: number of columns of the matrix. |
Return: |
S_OK: all is well. E_FAIL: failed. |
Note: |
|
Method: |
StVariableIndex(BSTR variableName, LONG *variableIndex) |
---|---|
Description: |
Get the index of a Stata variable. |
Input: |
variableName: the variable name. |
Output: |
variableIndex: variable index if the result is greater than 0, error code if the result is less than 0. |
Return: |
S_OK: all is well. |
Note: |
|
Method: |
VariableLabel(BSTR variableName, BSTR *variableLabel) |
---|---|
Description: |
Get the label of a variable. |
Input: |
variableName: the variable name. |
Output: |
variableLabel: the variable label. |
Return: |
S_OK: all is well |
Note: |
|
Method: |
VariableLabelArray(VARIANT *variableLabels) |
---|---|
Description: |
Get the labels of all variables. |
Input: |
None. |
Output: |
variableLabels: a string array of all variable labels. |
Return: |
S_OK: all is well. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
If variable i does not have label, variableLabels[i−1] is an empty string. |
Method: |
VariableLabelAsVariants(VARIANT *variableLabels) |
---|---|
Description: |
Get the labels of all variables. |
Input: |
None. |
Output: |
variableLabels: an array of variants. |
Return: |
S_OK: all is well. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
If variable i does not have label, variableLabels[i−1] is an empty string. The method can be used in VBSCript. |
Method: |
StVariableName(LONG variableIndex, BSTR *variableName) |
---|---|
Description: |
Get the name of a variable. |
Input: |
variableIndex: the variable index. |
Output: |
variableName: the variable name. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: input argument is out of range. |
Note: |
The input argument must satisfy 1≤variableIndex≤ nvar. Otherwise, the method returns a NULL string and produces an INVALID_PARAMETER error. |
Method: |
VariableNameArray(VARIANT *variableNames) |
---|---|
Description: |
Get the names of all variables. |
Input: |
None. |
Output: |
variableName: a string array of all variable names. |
Return: |
S_OK: all is well. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
Stata variable index starts from 1. The string array returned from the method starts from 0. Hence, the first variable’s name is in variableName[0]. |
Method: |
VariableNameAsVariants(VARIANT *variableNames) |
---|---|
Description: |
Get the names of all variables. |
Input: |
None. |
Output: |
variableName: an array of variants. |
Return: |
S_OK: all is well. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
Stata variable index starts from 1. The string array returned from the method starts from 0. Hence, the first variable’s name is in variableName[0]. The method can be used in VBSCript. |
Method: |
VariableType(BSTR variableName,BYTE *variableType) |
---|---|
Description: |
Get the data type of a variable. |
Input: |
variableName: the variable name. |
Output: |
A byte represents the variable data type. The stata.StataVariableTypes enum type has all possible data type values. They are vstUndef: type not defined |
Return: |
S_OK: all is well. |
Note: |
The method returns 0, which is the same as vstUndef if the variable cannot be found. |
Method: |
VariableTypeArray(VARIANT *variableTypes) |
---|---|
Description: |
Get the data type array of all variables. |
Input: |
None. |
Output: |
variableTypes: a data type array of all variables. |
Return: |
S_OK: all is well. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
Method: |
VariableTypeAsVariants(VARIANT *variableTypes) |
---|---|
Description: |
Get the data type array of all variables. |
Input: |
None. |
Output: |
variableTypes: an array of variants. |
Return: |
S_OK: all is well. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The method can be used in VBScript. |
Method: |
VariableValLabel(LONG variableIndex, BSTR *valueLabelName) |
---|---|
Description: |
Get the value label name of a variable. |
Input: |
variableIndex: variable index. |
Output: |
valueLabelName: the variable value label name. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: invalid variable index. |
Note: |
1. The method returns an empty string if the variable has no value label. 2. The method returns a NULL string if the variable index is invalid. |
Method: |
VariableValLabelArray(VARIANT *valueLabels) |
---|---|
Description: |
Get the value labels of all variables. |
Input: |
None. |
Output: |
valueLabels: a string array of the variable value label names. |
Return: |
S_OK: all is well. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
If variable i does not have value label, valueLabels[i−1] is an empty string. |
Method: |
VariableValLabelAsVariants(VARIANT *valueLabels) |
---|---|
Description: |
Get the value labels of all variables. |
Input: |
None. |
Output: |
valueLabels: an array of variants. |
Return: |
S_OK: all is well. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
If variable i does not have value label, valueLabels[i−1] is an empty string. The method can be used in VBScript. |
Method: |
VariableValLabelCount(BSTR valueLabelName, LONG *count) |
---|---|
Description: |
Get the number of values in a value label. |
Input: |
valueLabelName: the value label name. |
Output: |
count/: the number of values in the value label. Possible numbers are −1: error or too many values |
Return: |
S_OK: all is well. |
Note: |
|
Method: |
VariableValLabelValueArray(BSTR valueLabelName, VARIANT *value) |
---|---|
Description: |
Get all values in a value label. |
Input: |
valueLabelName: the value label name. |
Output: |
value: an integer array of values in the value label. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: value label name is invalid. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The method returns an integer array of all the values in a value label. The method VariableValLabelLabelArray() returns a string array of all the labels in a value label. Matching values and labels at the same index in both arrays gives you all the value–label pairs in a value label. |
Method: |
VariableValLabelValueAsVariants(BSTR valueLabelName, VARIANT *value) |
---|---|
Description: |
Get all values in a value label. |
Input: |
valueLabelName: the value label name. |
Output: |
value: an array of values variants. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: value label name is invalid. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The method returns an array of variants of all the values in a value label. The method VariableValLabelLabelAsVariants() returns an array of variants of all the labels in a value label. Matching values and labels at the same index in both arrays give you all the value–label pairs in a value label. The method can be used in VBScript. |
Method: |
VariableValLabelLabelArray(BSTR valueLabelName, VARIANT *labels) |
---|---|
Description: |
Get all labels in a value label. |
Input: |
valueLabelName: the value label name. |
Output: |
labels: a string array of all labels in a value label. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: value label name is invalid. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
Method: |
VariableValLabelLabelAsVariants(BSTR valueLabelName, VARIANT *labels) |
---|---|
Description: |
Get all labels in a value label. |
Input: |
valueLabelName: the value label name. |
Output: |
labels: an array of variants. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: value label name is invalid. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The method can be used in VBScript. |
Method: |
VariableData(LONG varNo, LONG obsStart, LONG obsEnd, VARIANT *data) |
---|---|
Description: |
Return observations from obsStart to obsEnd for a variable. |
Input: |
varNo: the variable index. |
Output: |
data: a variant array. The array contains either a string array or a double array, depending on the data type of the variable. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: invalid variable index or observation index. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The input arguments must satisfy 1≤obsStart≤ obsEnd≤nobs and 1≤varNo≤nvar; otherwise, the method produces an ERROR_INVALID_PARAMETER error and returns a NULL array. |
Method: |
VariableDataAsVariants(LONG varNo, LONG obsStart, LONG obsEnd, VARIANT *data) |
---|---|
Description: |
Return observations from obsStart to obsEnd for a variable. |
Input: |
varNo: the variable index. |
Output: |
data: an array of variants. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: invalid variable index or observation index. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The input arguments must satisfy 1≤obsStart≤ obsEnd≤nobs and 1≤varNo≤nvar; otherwise, the method produces an ERROR_INVALID_PARAMETER error and returns a NULL array. The method can be used in VBSCript. |
Method: |
VariableDataFromName(BSTR variableName, ULONG obsStart, ULONG obsEnd, VARIANT *data) |
---|---|
Description: |
Return observations from obsStart to obsEnd for a variable. |
Input: |
variableName: name of the variable. |
Output: |
dataSet: a variant array that contains either a string array or a double array, depending on the data type of the variable. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: invalid variable or invalid observation index. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The input arguments must satisfy 1≤oStart≤ oEnd≤nobs; otherwise, the method throws ERROR_INVALID_PARAMETER. |
Method: |
VariableDataFromNameAsVariants(BSTR variableName, ULONG obsStart, ULONG obsEnd, VARIANT *data) |
---|---|
Description: |
Return observations from obsStart to obsEnd for a variable. |
Input: |
variableName: name of the variable. |
Output: |
dataSet: a array of variants. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: invalid variable or invalid observation index. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The input arguments must satisfy 1≤oStart≤ oEnd≤nobs; otherwise, the method throws ERROR_INVALID_PARAMETER. The method can be used in VBScript. |
Method: |
VariablesData(ULONG vStart, ULONG vEnd, ULONG oStart, ULONG oEnd, VARIANT *data) |
---|---|
Description: |
return observations from oStart to oEnd for variables from vStart to vEnd. |
Input: |
vStart: start variable index. |
Output: |
data: a variant array in which ith element contains a string or a double array, depending on the data type of the (i+vStart)th variable. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: invalid variable index or observation index. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The input arguments must satisfy 1≤vStart≤vEnd≤nvar and 1≤oStart≤oEnd≤nobs; otherwise, the method produces an ERROR_INVALID_PARAMETER error and returns a NULL array. |
Method: |
VariablesDataAsVariants(ULONG vStart, ULONG vEnd, ULONG oStart, ULONG oEnd, VARIANT *data) |
---|---|
Description: |
return observations from oStart to oEnd for variables from vStart to vEnd. |
Input: |
vStart: start variable index. |
Output: |
data: an array of variants. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: invalid variable index or observation index. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The input arguments must satisfy 1≤vStart≤vEnd≤nvar and 1≤oStart≤oEnd≤nobs; otherwise, the method produces an ERROR_INVALID_PARAMETER error and returns a NULL array. The method can be used in VBScript. |
Method: |
VariablesAllData(ULONG oStart, ULONG oEnd, VARIANT *data) |
---|---|
Description: |
return observations from oStart to oEnd for all variables. |
Input: |
oStart: start observation index. |
Output: |
data: a variant array in which ith element contains a string or a double array, depending on the data type of the variable. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: invalid variable index or observation index. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The input arguments must satisfy 1≤oStart≤oEnd≤nobs; otherwise, the method produces an ERROR_INVALID_PARAMETER and returns a NULL array. |
Method: |
VariablesAllDataAsVariants(ULONG oStart, ULONG oEnd, VARIANT *data) |
---|---|
Description: |
return observations from oStart to oEnd for all variables. |
Input: |
oStart: start observation index. |
Output: |
data: an array of variants in which each element is an array of variants. |
Return: |
S_OK: all is well. ERROR_INVALID_PARAMETER: invalid variable index or observation index. ERROR_OUTOFMEMORY: system out of memory. |
Note: |
The input arguments must satisfy 1≤oStart≤oEnd≤nobs; otherwise, the method produces an ERROR_INVALID_PARAMETER error and returns a NULL array. The method can be used in VBSCript. |
Method: |
UtilIsStataFree(LONG *status) |
---|---|
Description: |
Check whether Stata has more commands to execute. |
Input: |
None. |
Output: |
status:
TRUE: Stata has no more commands to execute. |
Return: |
S_OK: all is well. |
Note: |
The method can be used as a polling method with DoCommandAsync(). |
Method: |
UtilIsStataFreeEvent() |
---|---|
Description: |
Check whether Stata has more commands to execute. If it does send an OnFinish event back to the client. |
Input: |
None. |
Output: |
None. |
Return: |
S_OK: all is well. |
Note: |
The method files a work thread to monitor the status of Stata. It runs the OnFinish event in the Stata main thread after the work thread returns. |
Method: |
UtilIsStMissingValue(DOUBLE value, VARIANT_BOOL *bMissing) |
---|---|
Description: |
Check whether a number will be treated as missing in Stata. |
Input: |
value: the number to be checked. |
Output: |
bMissing:
True if the value is greater than or equal to the Stata missing
value. |
Return: |
S_OK: all is well. |
Note: |
The method requires a round trip from the client to Stata for the value to be checked. If there are many numbers to be checked, you should use the next method, UtilGetStMissingValue(), to get the Stata missing value and do the checking in the client. |
Method: |
UtilGetStMissingValue(DOUBLE *misVal) |
---|---|
Description: |
Get the number that represents the Stata missing value. |
Input: |
None. |
Output: |
misVal: the Stata missing value. |
Return: |
S_OK: all is well. |
Note: |
Method: |
UtilSetStataBreak() |
---|---|
Description: |
set Stata break key to stop a lengthy Stata command execution. |
Input: |
None. |
Output: |
None. |
Return: |
S_OK: all is well. |
Note: |
Method: |
UtilShowStata(USHORT mode) |
---|---|
Description: |
Control the Stata display mode from client. |
Input: |
mode: Stata display mode. Possible values are
1: hide Stata |
Output: |
None. |
Return: |
S_OK: all is well. |
Note: |
DoCommand() behaves differently when Stata is hidden. |
Method: |
UtilStataErrorCode(LONG *returnCode) |
---|---|
Description: |
Get the return code. |
Input: |
None. |
Output: |
returnCode: return code stored in Stata _rc. |
Return: |
S_OK: all is well. |
Note: |